
Download Source Code
Introduction
A splash screen is a window that displays copyright information while your application initializes itself. To see an example of this, just fire up Visual Studio 2010.
A splash screen is not always desirable. Some users may be annoyed that they must wait to use their software. However, if you make your splash screen interesting and don't display it for too long, it's unlikely to annoy anyone, especially if your app takes a little while to initialize anyway.
In this article, I'll present a fun splash screen that has the shape of something being splashed against a surface. This makes the splash screen more fun, and also presents some interesting technical issues.
Non-Rectangular Windows
My splash screen is a regular Windows form. We just need to take a few steps to make it non-rectangular.
The first step is to create a bitmap. The bitmap serves both as the background of the splash window, and also to define the shape of that window. Here's the image I created. Note the magenta region outside of the splash image. This will represent the transparent area of the form.
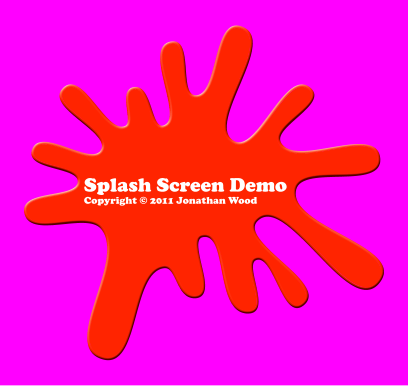
Next, set the form's FormBorderStyle property to None. Note that this setting also removes the form's title bar. Without a title bar, the user cannot move, resize or close the form unless you add controls and code to support this. In the case of our splash screen, the form will close automatically and we don't want the user manipulating the form while it's open. So this works just fine.
Next, set the form's BackgroundImage property to the bitmap created for your splash screen. More than likely, you'll also want to set the form's BackgroundImageLayout to Center. Be sure to size the form so that the bitmap covers the entire background. If the bitmap does not extend to the edges of the form, those edges will not be transparent.
You also need to set the form's TransparencyKey property to the exact color of the transparency area in your bitmap. In my bitmap, that color is Magenta. Note that you can't have any other part of your bitmap this same color or that area will also appear transparent.
That's all there is to that. If you run the project, the form should take on the shape of the non-transparent area of your bitmap.
Automatically Closing the Splash Screen
Now that the splash screen looks how we want, we need to program its behavior. Specifically, we want the splash screen to appear on top of any other form, and then close after a few seconds.
The splash screen should not block initialization of the application. In an ideal situation, the splash screen would appear while the application initializes so that it doesn't at all delay the time until the user can start using it. So, for example, we shouldn't display the form using ShowDialog() because the application couldn't do anything else while the splash screen was visible (unless we have the form call code in another form, which complicates the design).
Listing 1 shows my code for the splash screen form. It has a public method, ShowSplash(), to handle the details of showing the form. This method takes two arguments, the duration the splash screen should be visible (in milliseconds), and the owner window. The owner window argument should refer to the application's main start-up form. This argument is passed to the Show() method. Because the splash screen window is owned by the other window, the splash screen will always appear on top of that window.
After the window has been displayed, the code programs the timer to fire the Tick event after the specified duration. At this point, ShowSplash() returns to allow the application to continue intializing.
Listing 1: Code for the Splash Screen Form
public partial class Splash : Form
{
public Splash()
{
InitializeComponent();
}
/// <summary>
/// Displays the splash screen for the given duration on top
/// of the specified owner window.
/// </summary>
/// <param name="duration">Duration in milliseconds</param>
/// <param name="owner">Owner window</param>
public void ShowSplash(int duration, IWin32Window owner)
{
Show(owner);
timer1.Interval = duration;
timer1.Enabled = true;
}
private void timer1_Tick(object sender, EventArgs e)
{
Close();
}
}
Using the Splash Screen
At this point, using the splash screen is very easy. In the Load event handler, the main form simply creates an instance of the Splash form and calls the ShowSplash() method. Listing 2 demonstrates this.
Listing 2: Code that Calls the Splash Screen
private void Form1_Load(object sender, EventArgs e)
{
Splash frm = new Splash();
frm.ShowSplash(4000, this);
}
Conclusion
I should point out that the main form (if visible) is still active while the splash screen is displayed. This means, for example, that you can interact with any controls on the main that the splash screen does not cover. This may or may not be an issue for you.
If it is an issue, possible workarounds include disabling the main form or going ahead and using ShowDialog() to display the splash screen, and adding the necessary hooks that allow the application to continue initializing while the splash screen is visible.
The attached demo project contains all my source code and bitmap. Of course, you'll want to customize the splash screen for your own application, but the code should give you a good start.
End-User License
Use of this article and any related source code or other files is governed
by the terms and conditions of
.
Author Information
Jonathan Wood
I'm a software/website developer working out of the greater Salt Lake City area in Utah. I've developed many websites including Black Belt Coder, Insider Articles, and others.